We are proud to annouce that STRIP for Android, the secure password manager and data vault, is now available in the Google Play store!
STRIP has been protecting data on mobile devices since 1998, and we've continued to evolve the product over the years to run on iOS, Mac, Windows, and now Android. Like its peers, STRIP for Android has many advanced features and the best security available:
STRIP for Android
- A flexible data format that allows you to organize your information how you want into categories, entries and fields (you can even create your own labels and specify their data type)
- Full text search, providing quick access to find data where ever you are in the application
- Synchronization via Dropbox and replication out to STRIP for Windows and STRIP for OSX (also ensuring that a reliable data backup is always available)
- A secure random password generator
- Assignment of icons to entries and categories for easy visual identification
- Launch websites, dial phone numbers and address emails
Most importantly, STRIP for Android features complete data encryption, protecting your most valuable information with SQLCipher. An open source extension to SQLite, SQLCipher uses 256-bit AES to encrypt the entire application database. We began the process of porting SQLCipher to Android late last year in conjunction with The Guardian Project to ensure a stable and secure platform, before even starting work on STRIP for Android. SQLCipher has been independently reviewed by many security professionals; for instance Elcomsoft presented findings about STRIP at the BlackHatEU conference, concluding that the application backed by SQLCipher was the "the most resilient to password cracking".
If you are looking for a password and data manager on the Android platform that provides a high degree of security and user flexibility, check out STRIP for Android and let us know what you think.
We mentioned awhile back that we were busy working on a port of Strip for the Android platform. Development has been coming along smoothly and we launched a beta testing program to get feedback from some of our most enthusiastic users which has been very successful. Below you can see what some of the interface looks like:

If you are interested in using the same technology that was identified in the most resilient application to password cracking at the BlackHatEU conference on the Android platform be sure to sign up here to be notified when Strip for Android is released.
Following up to my earlier post about improving the security of the ASP.NET SqlMembershipProvider, and to Troy Hunt's excellently thorough article Our Password Hashing Has No Clothes and further discussions with @thorsheim, @blowdart, and @klingsen (all of whom I recommend following), I took a second look at how to use the algorithms in the Zetetic.Security package without mucking about with machine.config and the .NET Global Assembly Cache, which really complicate the deployment picture.
The bad news is that the .NET base class libraries only read "name-to-algorithm" mappings from machine.config. I was pretty surprised to see this, but it's plain as day in System.Security.Cryptography.CryptoConfig.OpenCryptoConfig().
The good news is that adding to the HashAlgorithms an application can use is super, super easy. Start by grabbing the Zetetic.Security package from NuGet; next, one line of code will do the trick (in Global.asax's Application_Start, for example):
System.Security.Cryptography.CryptoConfig.AddAlgorithm(
typeof(Zetetic.Security.Pbkdf2Hash),
"pbkdf2_local");
Adjust the membership settings in Web.config as per usual:
<membership hashAlgorithmType="pbkdf2_local"><!-- other stuff --><membership>
Voila, your ASP.NET application is now using a much, much stronger password hash algorithm than the (really rather embarrassing) defaults of SHA1 and SHA256.
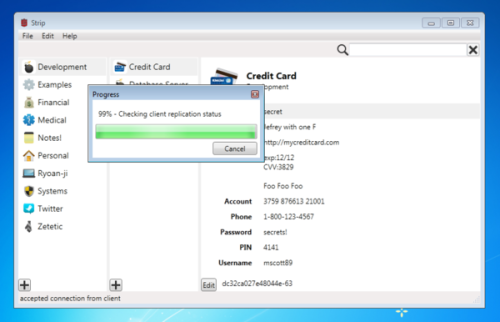
Starting now until July 6th, STRIP Password Manager for Windows is on sale, just enter the discount code STRIPHAPPY4TH at check-out for 25% off. If you've been using STRIP on your iPhone and you want reliable backups, and a desktop editor, STRIP for Windows is for you.
As mobile development continues to grow at a rapid pace, the increased need for developers to take advantage of their existing expertise in programming languages grows with it. To this point, we've ported SQLCipher to run on both MonoTouch and Mono for Android. You can now develop .NET applications, secured by SQLCipher running on both iOS and Android platforms. We have prepared licensed binaries for sale here and have tutorials on integrating SQLCipher for MonoTouch as well as SQLCipher on Mono for Android. If you've been looking for a good way to secure your data on major mobile platforms running .NET with SQLCipher we now have the solution. Take a look!